Schaltungaufbau
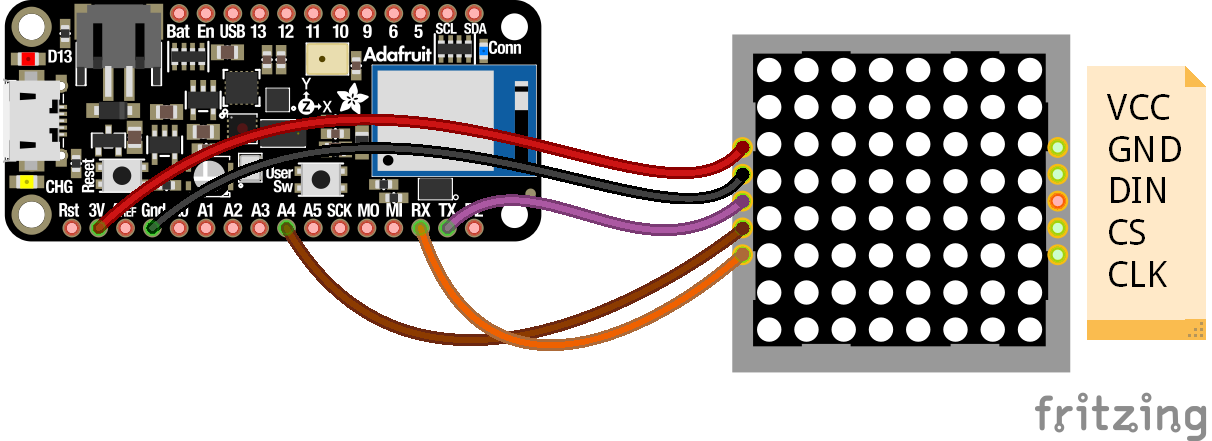
Codebeispiele
Beispiel 1
from adafruit_max7219 import matrices
import board
import busio
import digitalio
import time
# Maxtrixdisplay initialisieren
cs = digitalio.DigitalInOut(board.A4)
spi = busio.SPI(board.RX, MOSI=board.TX)
matrix = matrices.Matrix8x8(spi, cs)
while True:
matrix.brightness(3)
matrix.fill(1)
matrix.pixel(3, 3)
matrix.pixel(3, 4)
matrix.pixel(4, 3)
matrix.pixel(4, 4)
matrix.show()
time.sleep(1.0)
matrix.scroll(-1, -1)
matrix.show()
time.sleep(1.0)
matrix.clear_all()
s = 'Hello, World!'
for c in range(len(s)*8):
matrix.fill(0)
matrix.text(s,-c,0)
matrix.show()
time.sleep(0.05)
Beispiel 2
from adafruit_max7219 import matrices
import board
import busio
import digitalio
import time
# Maxtrixdisplay initialisieren
cs = digitalio.DigitalInOut(board.A4)
spi = busio.SPI(board.RX, MOSI=board.TX)
matrix = matrices.Matrix8x8(spi, cs)
# Alle LED-Pixel ausschalten
matrix.fill(0)
matrix.show()
# Pause zwischen den Bildern
frame_delay = 0.5
# Aktuelle Bildnummer
bild_nummer = 0
# Bilder der Animation als Liste von Listen von Listen
animation = [
# frame 0 - alien #1 frame 1
[
[0, 0, 0, 1, 1, 0, 0, 0],
[0, 0, 1, 1, 1, 1, 0, 0],
[0, 1, 1, 1, 1, 1, 1, 0],
[1, 1, 0, 1, 1, 0, 1, 1],
[1, 1, 1, 1, 1, 1, 1, 1],
[0, 0, 1, 0, 0, 1, 0, 0],
[0, 1, 0, 1, 1, 0, 1, 0],
[1, 0, 1, 0, 0, 1, 0, 1]
],
# frame 1 - alien #1 frame 2
[
[0, 0, 0, 1, 1, 0, 0, 0],
[0, 0, 1, 1, 1, 1, 0, 0],
[0, 1, 1, 1, 1, 1, 1, 0],
[1, 1, 0, 1, 1, 0, 1, 1],
[1, 1, 1, 1, 1, 1, 1, 1],
[0, 0, 1, 0, 0, 1, 0, 0],
[0, 1, 0, 1, 1, 0, 1, 0],
[0, 1, 0, 0, 0, 0, 1, 0]
]
]
while True:
for x in range(8):
for y in range(8):
matrix.pixel(x, y, animation[bild_nummer][x][y])
# next animation frame
bild_nummer = bild_nummer + 1
# show animation
matrix.show()
# pause for effect
time.sleep(frame_delay)
if bild_nummer == len(animation):
bild_nummer = 0
Beispiel 3 - Snake Spiel
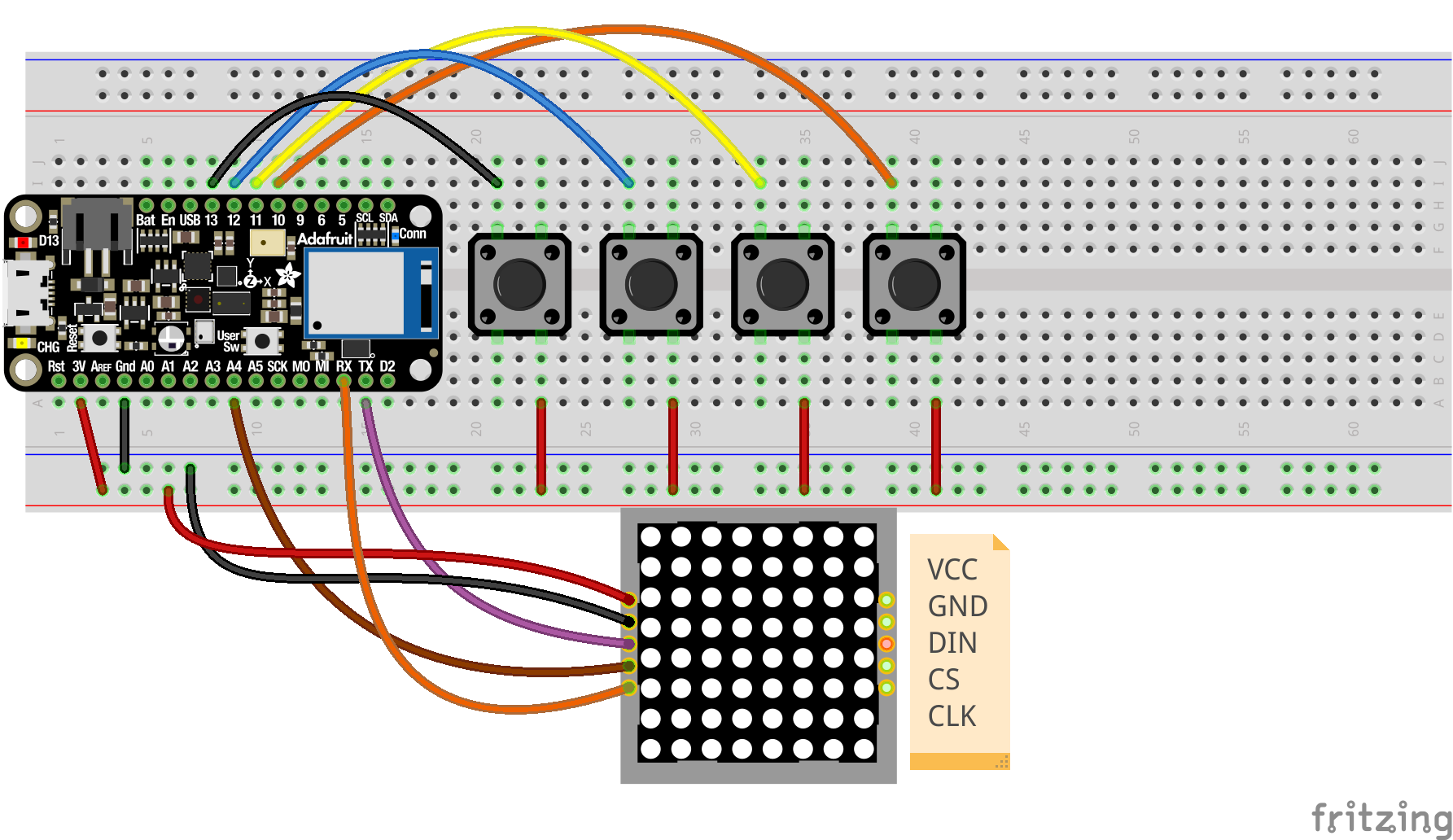
1from adafruit_max7219 import matrices
2import board
3import busio
4import digitalio
5from adafruit_debouncer import Debouncer
6import time
7import random
8
9button1 = digitalio.DigitalInOut(board.D10)
10button2 = digitalio.DigitalInOut(board.D11)
11button3 = digitalio.DigitalInOut(board.D12)
12button4 = digitalio.DigitalInOut(board.D13)
13button1.switch_to_input(pull=digitalio.Pull.DOWN)
14button2.switch_to_input(pull=digitalio.Pull.DOWN)
15button3.switch_to_input(pull=digitalio.Pull.DOWN)
16button4.switch_to_input(pull=digitalio.Pull.DOWN)
17button1_d = Debouncer(button1)
18button2_d = Debouncer(button2)
19button3_d = Debouncer(button3)
20button4_d = Debouncer(button4)
21
22# Maxtrixdisplay initialisieren
23cs = digitalio.DigitalInOut(board.A4)
24spi = busio.SPI(board.RX, MOSI=board.TX)
25matrix = matrices.Matrix8x8(spi, cs)
26
27# Maximale Helligkeit
28matrix.brightness(3)
29# Alle LED-Pixel ausschalten
30matrix.clear_all()
31matrix.show()
32
33# Eine Liste speichert die Koordinaten der Schlangensegmente.
34# Erstes Element ist der Kopf.
35snake = [(3,3),(3,4)]
36direction = (0,-1)
37foods = []
38maxfood = 4
39
40def drawSnake():
41 for segment in snake:
42 matrix.pixel(segment[0],segment[1], 1)
43 matrix.show()
44
45def moveHead(x, y):
46 global direction
47 (oldhead_x, oldhead_y) = snake[0]
48 newhead = ((oldhead_x + x) % 8, (oldhead_y + y) % 8)
49
50 for segment in snake:
51 if segment == newhead: # Game Over
52 gameOver()
53 return
54
55 direction = (x, y)
56 snake.insert(0, newhead)
57 matrix.pixel(newhead[0],newhead[1], 1)
58 matrix.show()
59
60 if not eatFood():
61 removeTail()
62
63
64def removeTail():
65 tail = snake.pop()
66 matrix.pixel(tail[0], tail[1], 0)
67 matrix.show()
68
69def eatFood():
70 for food in foods:
71 if snake[0] == food:
72 foods.remove(food)
73 return True
74 return False
75
76def newFood():
77 x = random.randint(0, 7)
78 y = random.randint(0, 7)
79 for segment in snake:
80 if segment == (x, y):
81 return
82 if len(foods) >= maxfood:
83 return
84 foods.append((x,y))
85 matrix.pixel(x, y, 1)
86 matrix.show()
87
88def gameOver():
89 global snake
90 global direction
91 global food
92
93 matrix.clear_all()
94 s = 'Game Over!'
95 for c in range(len(s)*8):
96 matrix.fill(0)
97 matrix.text(s,-c,0)
98 matrix.show()
99 time.sleep(0.05)
100 matrix.clear_all()
101
102 snake = [(3,3),(3,4)]
103 direction = (0, -1)
104 food = []
105 drawSnake()
106 lastFood = time.monotonic()
107 lastMove = time.monotonic()
108
109
110lastFood = time.monotonic()
111lastMove = time.monotonic()
112drawSnake()
113
114while True:
115 button1_d.update()
116 button2_d.update()
117 button3_d.update()
118 button4_d.update()
119
120 if button1_d.rose:
121 direction = (0, 1)
122
123 if button2_d.rose:
124 direction = (1, 0)
125
126 if button3_d.rose:
127 direction = (-1, 0)
128
129 if button4_d.rose:
130 direction = (0, -1)
131
132 if (time.monotonic() - lastFood) > 5:
133 newFood()
134 lastFood = time.monotonic()
135
136 if (time.monotonic() - lastMove) > 0.3:
137 moveHead(*direction)
138 lastMove = time.monotonic()
139
Du hast noch nicht genug vom Thema?
Hier findest du noch weitere passende Inhalte zum Thema: